Task-2-Data-Structures
intermediate Level
See Home Page
See Task 1 Page
See Task 3 Page
See Information Page
There are four files in this task: ArrayExample, ComicBooks, HashMapExercise, and ListExample.
It shows basic functions on how to add more values, and how to create them.
The purpose of this task is to show how data structures are used.
A variable is a container to hold a value that can change.
A data structure like an array can hold muliple variables.
Two coding tasks have been given: HashMapExercise, and ComicBook. Comic book uses a hash map to store ‘quality’ with a ‘price’ as key-value pairs.It also uses an enumeration which are like constants.
Remember to save your work and to check the feedback provided under this document.
Keywords
Match the keywords with the definitions: (copy and paste)
- Data Structure
- Array
- element
- Storage
- List
-
Map
- A set of data values of the same type, stored in a sequence in a computer program.
- contains values on the basis of key, i.e. key and value pair. Each key and value pair is known as an entry. Contains unique keys.
- The way that data is stored in a database or program.
- An individual component of an array.
- found in the java.util package and inherits the Collection interface.
Has the facility to maintain the ordered collection. It contains the index-based methods to insert, update, delete and search the elements.
It can have the duplicate elements also. We can also store the null elements in the list.
Predict the output
int[] myNum = {10, 20, 30, 40};
System.out.println(myNum[1]);
- Guess what the output is from the code above:
- Actual output:
int[][] myNumbers = { {1, 2, 3, 4}, {5, 6, 7} }; //2D array
int x = myNumbers[1][2];
System.out.println(x);
- Guess what the output is from the code above:
- Actual output:
ArrayList<Integer> myNum = new ArrayList<>();
myNum.add(20);
myNum.add(10);
myNum.add(40);
myNum.add(30);
Collections.sort(myNum);
System.out.println(myNum.get(3));
- Guess what the output is from the code above:
- Actual output: See answer here
Introductory Steps:
- Read everything above.
- Keep this tab open, and open Gitpod
- Familiarise yourself with the arrayExample and listExample. Look at how they are created.
- Run the code. Then try to change some numbers around.
- Optional: Add some extenstions to it: Make a bubble sort, or create another array/list, Search for specific values etc
HashMapExercise.java Steps:
Optional: ComicBooks.java
Not currently marked
Tips/Hints
- challenge 1 hint: if hMap.get(i) == …….
- empty values can be “” -
- Look at the put methods to insert data
- use the get method to retrieve values from a hash map
- Name the HashMap to what the rest of the code calls from.
- Optional task 3 NOTE: Remember you have to change the size of the comix array.)
If you are ever stuck, ask a friend and look at the examples. While they may be different data structures they handle data very similarly.
Look at w3schools too.
Questions to think about:
- Which data structures are appropriate for what kinds of tasks?
- Do they have varying speeds?
Save your work
- Remember to save your work. You can see your progress on the links as well by saving.
- To save your work, enter submit to the command line.
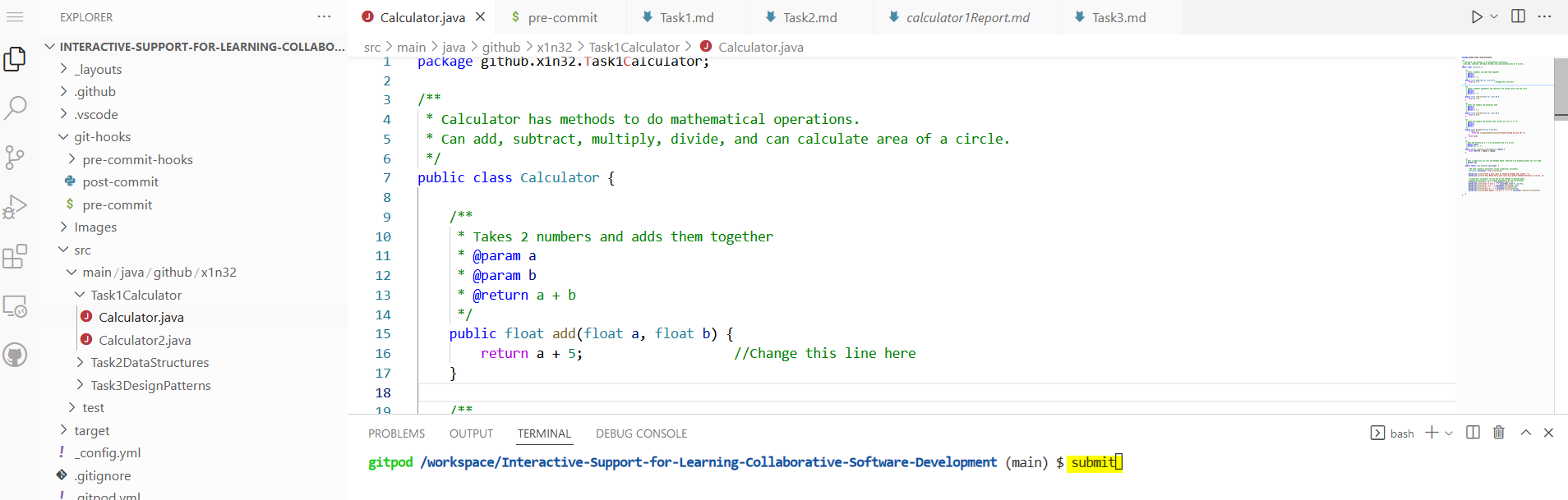
Hash Map Task status:
See report on Hash Map Task
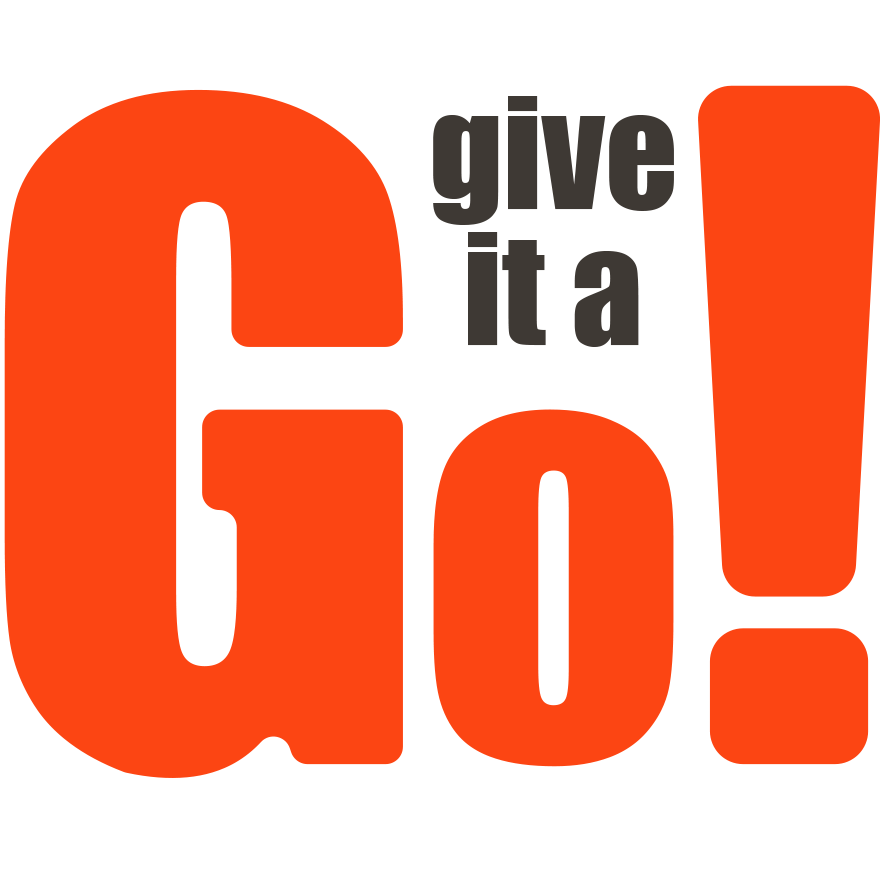
Answers are in the ‘answer’ branch.